Managing configuration settings in .NET applications offers numerous possibilities, yet often I find
the available documentation to be less than ideal. This makes it challenging to identify the beste™
or at least a user-friendly method. In this article, I will outline how I have approached this
issue.
The manual method
Numerous guides, both on the internet and on the Microsoft website, explain how to access
configuration data using the
class in C#. This data is provided through JSON, environment variables, and startup arguments.
Often, this data is manually retrieved.
The problem with this method is its susceptibility to errors. On one hand, it involves handling
strings that do not provide type safety, and on the other hand, it can become particularly
complicated when more complex data than simple strings is needed.
Complex data structures
The following JSON file is designed to be practical and includes elements that can cause headaches
during manual parsing: various data types, including enumerations, POCOs and arrays.
This data structure, when implemented in C#, would look something like this:
Reading this structure can be extremely tedious (Pain in the Ass®). It's worth noting that in
this example,
particularly stands out as it causes the application to trigger the HTTP status code
without any apparent reason. This is a brilliant idea that should be implemented somewhere in every
application.
Automatic Mapping
The mapping process can be simplified by using the
class from the
.
package. This approach involves deriving from the class and defining a lambda expression in the
constructor. This delegate receives an instance of the DTO class as a parameter. Since the
expression is specified in the constructor, an instance of
is being captured and also in the scope. The
method is then used to pass the path specified by a string (separated by :) in the configuration tree along with the instance of the options class, thereby writing the values into the object.
To inform the Dependency Injection (DI) system that this options class is available, it is added to
the
.
After this step, the class becomes available to other classes registered in the Dependency Injection
(DI) container.
Advanced configuration
Since the container is already available at the time of setup, it is possible to request other
services if specific actions are necessary for the proper construction of the options class. This
provides flexibility and enhanced possibilities for customization.
In the next article,
I will demonstrate how to use
to respond to changes in the configuration within the program. This allows for real-time
updates, ensuring that your application can adapt dynamically to configuration modifications.
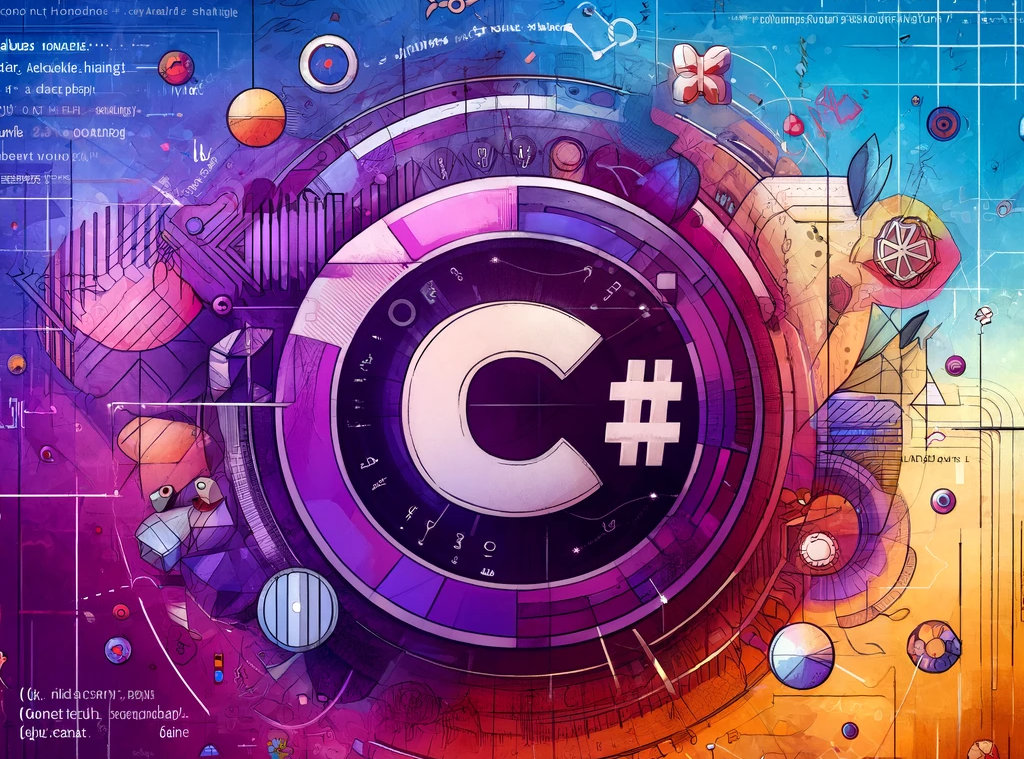
React to configuration changes in .NET
OptionsMonitoris a powerful tool that allows you to dynamically monitor configuration options and react to changes.