In this example, we consider the Starfleet's warp drive, which will be commissioned a few years
after 2063. Undoubtedly, the system must be informed if the chief engineer has made some changes
to the operating parameters.
Request
from the DI container.
Instead of using
rom the DI container, one should alternatively request
.
This interface provides the capability to register a delegate as soon as the configuration source
(often a JSON file) changes.
Add the JSON file to the
.
The JSON file is registered, specifying
to indicate that the file should be reloaded when changes are detected in the file system.
A glue to bind them all
In addition to setting up the options class using the
this article
, an
must also be registered. The
implementation is suitable for this purpose, as it handles the notifications.
class, which I examine in more detail in
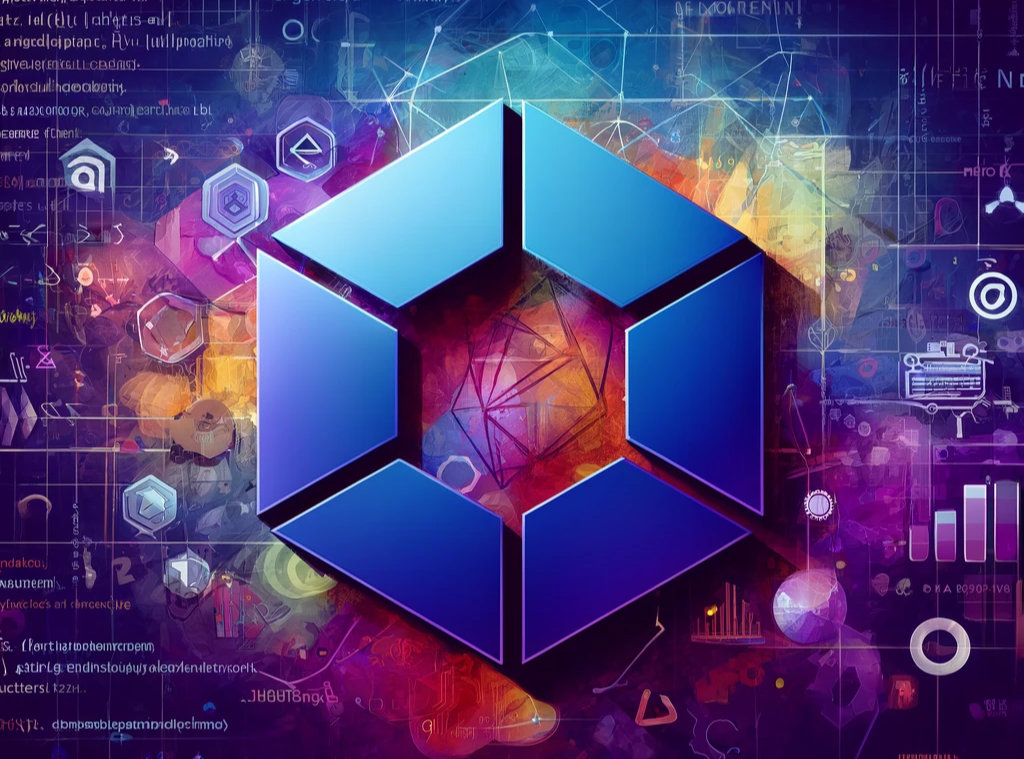
Read configuration data in .NET
Automatic parsing of configuration data from JSON into a DTO using the
ConfigureOptionsclass.